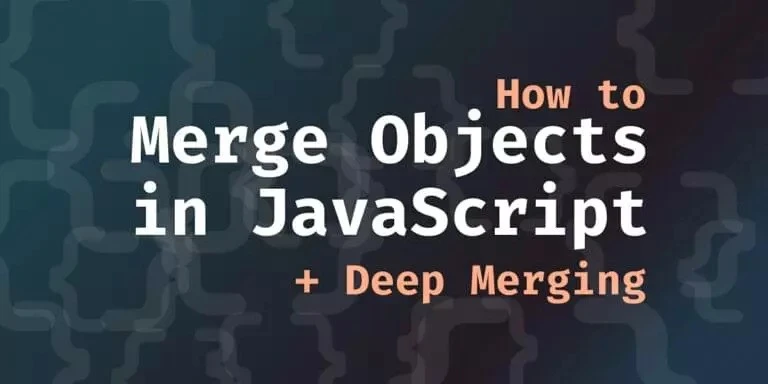
Tips on How to Join Objects in JavaScript
Different Ways to Combine Objects
1. The operator for spread out
In JavaScript, the spread operator (…) is often used to combine objects. This is what it looks like: {…object1,…object2}. If two source objects have properties with the same keys, the spread operator replaces the values in the target object with the most recent values from the source object.
const defaults = { color: 'red', size: 'medium' };
const userSettings = { color: 'blue' };
const combinedSettings = { ...defaults, ...userSettings };
console.log(combinedSettings);
// Output: { color: 'blue', size: 'medium' }
2. The give() method for objects
The JavaScript method Object.assign() can be used to combine items. The code for it is Object.assign(target, source1, source2,…), and it merges source objects into the target object. When two source objects have properties with the same keys, Object.assign() changes the values in the target object to the most recent values from the source object.
const defaults = { color: 'red', size: 'medium' };
const userSettings = { color: 'blue' };
const combinedSettings = Object.assign({}, defaults, userSettings);
console.log(combinedSettings);
// Output: { color: 'blue', size: 'medium' }
Problems and Things to Think About
Here are some problems that could happen when you use the spread operator or the Object.assign() method to combine objects in JavaScript:
1. Copying too little
When objects are merged, both the spread operator and Object.assign() do shallow copying. That is, objects that are nested are still references to the objects that they are nested inside. If you change the nested objects in the united object, it can affect the original objects, which could have effects you didn’t mean.
Check out Deep Merging below.
2. Properties that write over
Both the spread operator and Object.assign() replace the values in the new object with those from the most recent source object when they merge objects whose properties have the same keys. If you don’t treat this behaviour carefully, you could lose data.
3. Problems with compatibility
The spread operator is only available in ECMAScript 2015 (ES6). It can’t be used in older versions of JavaScript or in browsers like Internet Explorer. If your code needs to run in older settings, this could make things less compatible. It’s better to use Object.assign() instead, which works with more things.
4. Properties that can’t be listed
The spread operator and Object.assign() can only copy characteristics that can be listed from one object to another. Properties that can’t be listed aren’t copied during the joining process. This could leave data blank or cause strange behaviour.
5. Concerns about performance
Using Object.assign() or the spread operator may slow down your programme because they create new objects during the merging process. This can happen if you need to merge many objects or big objects.
6. Properties of a prototype
Object.assign() moves properties from the prototype of the source object to the target object. If the properties of the prototype of the source object conflict with the properties of the target object, this could cause strange behaviour. On the other hand, the spread operator doesn’t copy the features of the prototype.
When you use the spread operator or Object.assign() to merge objects in JavaScript, you need to be aware of these problems and dangers. To get around these problems, you might need to use different methods, like deep cloning or deep combining functions, in some situations.
Which One to Pick
The spread operator and Object.assign() can both be used to combine objects. The spread operator is shorter and more up-to-date, but Object.assign() works better with older JavaScript environments.
To pick a method, think about these things:
- If the spread operator works in your context, like with the latest version of ECMAScript, you should use it because the syntax is short.
- If you need to be compatible with older JavaScript environments, pick Object.assign().
- Read on to learn how to copy a stacked object, which is an object that has other objects inside it.
To deep copy and merge objects, use deep merging.
A shallow copy of the object(s) being copied is made by both the spread operator and Object.assign(). What this means is that the new object will not have copies of the nested objects (like arrays and functions), but will instead have links to the same ones.
Before merging things together, you need to know about this and avoid it.
The following example shows what can happen when you change stacked objects on a copied object:
const planet = {
name: 'Earth',
emoji: '🌍',
info: {
type: 'terrestrial',
moons: 1
}
};
// Shallow copy using the spread operator
const shallowCopyPlanet = { ...planet };
// Modifying the nested object in the shallow copy
shallowCopyPlanet.info.moons = 2;
console.log('Original planet:', planet.info.moons);
// Original planet: 2
console.log('Shallow copy of the planet:', shallowCopyPlanet.info.moons);
// Shallow copy of the planet: 2
The code’s result shows that the info.moons property on the original planet object has been changed by shallowCopyPlanet. This is probably not what you want to happen.
A custom method for deep merging
This function makes deep copies of several objects before merging them into one. It then gives the merged object. The code’s deepMergeObjects function takes in any number of objects, uses JSON.parse(JSON.stringify()) to make deep copies of them, and then uses the spread operator in the reduce() method to join them. The characteristics from the input objects will be deep copies in the merged object.
const deepMergeObjects = (...objects) => {
const deepCopyObjects = objects.map(object => JSON.parse(JSON.stringify(object)));
return deepCopyObjects.reduce((merged, current) => ({ ...merged, ...current }), {});
}
// Example usage:
const countries = {
USA: {
capital: 'Washington D.C.',
emoji: '🇺🇸',
population: 331000000
}
};
const countriesDetails = {
USA: {
language: 'English',
currency: 'USD'
},
Germany: {
capital: 'Berlin',
emoji: '🇩🇪',
language: 'German',
currency: 'EUR',
population: 83000000
}
};
const mergedData = deepMergeObjects(countries, countriesDetails);
In conclusion
Thank you for reading! I hope that this piece has taught you a lot about merging objects in JavaScript and not just the basics. Combining items in this should go well with your JavaScript skills and help you show off your coding skills. Join us at the SitePoint Community Forum if you have any questions or thoughts.
Insights into How to Merge Objects in JavaScript
What does it mean to “merge” items in JavaScript?
In JavaScript, merging objects means putting together two or more objects into a single type. This is often done to make it easier to handle and change data by combining properties and methods from different objects into one. All of the original objects’ values and methods will be in the merged object. If two or more features have the same value, the value from the most recent object will replace them.
How do I use the Spread operator to join items together in JavaScript?
In JavaScript, the Spread operator (…) is a modern and quick way to join things together. It lets an iterable, like a string or an array expression, be expanded in places where one or more arguments or parts are expected. Allow me to show you how to use it:
In this case, obj1 is set to { a: 1, b: 2 } and obj2 is set to { b: 3, c: 4 }. mergedObj is set to {…obj1,…obj2 } and the output is { a: 1, b: 3, c: 4 }.
What does the Object.assign() method do when you merge objects?
You can copy the values of all enumerable own properties from one or more source objects to a target object using the Object.assign() method. This will give you back the target item. It’s a great way to merge objects because it lets you turn multiple source objects into a single target object. It is important to remember that if more than one object has the same property, the value from the last object with that property will replace the values from the objects that came before it.
It’s possible to join nested objects in JavaScript.
As long as you use JavaScript, you can join nested objects. But the process is a little more complicated because you have to make sure that not only the top-level properties are merged properly, but also the properties of the objects that are inside the objects. A deep merge is another name for this. By default, neither Object.assign() nor the spread operator do a deep merge. To do this, you would have to make your own code or use a library like Lodash.
When you merge objects, what happens to features that are already there?
If you merge objects in JavaScript and there are duplicate properties, the value from the last object will replace the values from the objects that came before it. This is because when an object is merged, its features are taken from the source objects and put on the target object. If the target object already has a property with a value, the new value is put in that property’s place.
Do you know how to merge groups like you can with objects in JavaScript?
Yes, arrays can be combined in JavaScript using the same methods that are used for objects. Arrays are often joined together with the spread operator. This is an example:
The values of arr1 and arr2 are [1, 2, 3] and [4, 5, 6], respectively. The values of mergedArr are […arr1,…arr2] and […mergedArr]. The output is [1, 2, 3, 4, 5, 6].
How can I merge items without changing the ones that were already there?
When you merge objects with the spread operator or the Object.assign() method, they both make a new object and don’t change the original objects. Immutability is one of the most important ideas in functional programming, which means that data should never be changed. This is a key part of these methods.
In JavaScript, is it possible to combine items that have different types of data?
In JavaScript, you can join objects that store different kinds of data. No matter what kind of data the original objects were, the merged object will have all of their traits and methods. If there are multiple properties with different types of data, though, the value from the most recent object will replace the values from the earlier objects.
What happens to the speed when I merge objects in JavaScript?
In JavaScript, merging objects can slow things down, especially when you’re working with big objects. The time it takes to use both the spread operator and the Object.assign() method is O(n), where n is the number of values in the source objects. So, when you decide to merge your items, you should think about how big they are.
Is there a library in JavaScript that can help me merge objects?
Yes, there are a number of tools in JavaScript that can help you merge objects. One of the most famous is Lodash, which has many useful functions for working with objects and arrays, such as a merge function for combining objects deeply. jQuery is another library that lets you merge items with the $.extend() method.