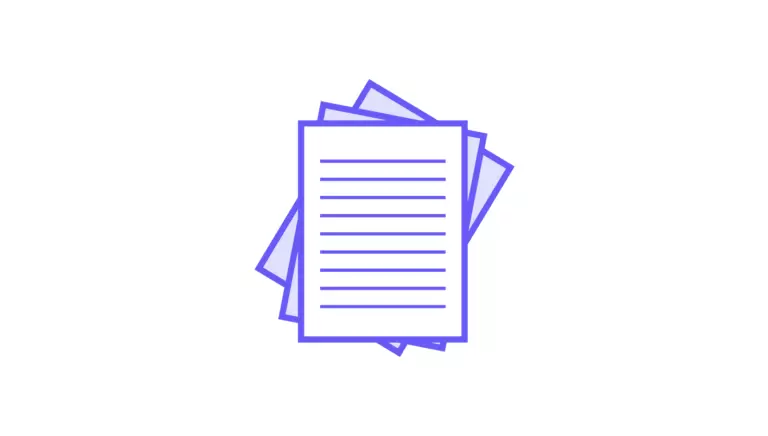
What you need to do to get URL parameters in JavaScript
This guide will teach you how to use JavaScript to read and change URL parameters.
How to Get a URL Parameter
This is a lot easier to do now that computers have the URLSearchParams interface. This sets up a bunch of useful ways to work with a URL’s query string.
If our URL is https://example.com/?product=shirt&color=blue&newuser&size=m, we can use window.location.search to get the query string:
const queryString = window.location.search;
console.log(queryString);
// ?product=shirt&color=blue&newuser&size=m
Then, we can use URLSearchParams to read the query string’s parameters:
const urlParams = new URLSearchParams(queryString);
In that case, we use the output to call any of its methods.
For instance, URLSearchParams.get() will give you the first number that goes with the given search parameter:
const product = urlParams.get('product')
console.log(product);
// shirt
const color = urlParams.get('color')
console.log(color);
// blue
const newUser = urlParams.get('newuser')
console.log(newUser);
// empty string
Other Methods That Can Help
Making sure that a parameter is present
You can use URLSearchParams.has() to see if a certain argument is present:
console.log(urlParams.has('product'));
// true
console.log(urlParams.has('paymentmethod'));
// false
Getting All of the Values of a Parameter
console.log(urlParams.getAll('size'));
// [ 'm' ]
//Programmatically add a second size parameter.
urlParams.append('size', 'xl');
console.log(urlParams.getAll('size'));
// [ 'm', 'xl' ]
You can get all the numbers that go with a certain parameter by calling URLSearchParams.getAll():
Going through the parameters
const
keys = urlParams.keys(),
values = urlParams.values(),
entries = urlParams.entries();
for (const key of keys) console.log(key);
// product, color, newuser, size
for (const value of values) console.log(value);
// shirt, blue, , m
for(const entry of entries) {
console.log(`${entry[0]}: ${entry[1]}`);
}
// product: shirt
// color: blue
// newuser:
// size: m
URLSearchParams also has some well-known Object iterator methods that let you go through its keys, values, and entries:
Help for Browsers
It’s good that browsers accept URLSearchParams. At the time this was written, all major browsers could handle it.
URLSearchParams
The URLSearchParams interface defines utility methods to work with the query string of a URL.
IE | Edge | Firefox | Chrome | Safari | iOS Safari | Opera Mini | Chrome for Android | Android Browser | Samsung Internet |
---|---|---|---|---|---|---|---|---|---|
9 | 121 | 123 | 122 | 17.2 | 17.2 | 4.4 | 22 | ||
10 | 122 | 124 | 123 | 17.3 | 17.3 | 4.4.4 | 23 | ||
11 | 123 | 125 | 124 | 17.4 | 17.4 | all | 123 | 123 | 24 |
126 | 125 | 17.5 | 17.5 |
Global: 97.38% + 0.01% = 97.39%
If you need to support old platforms like Internet Explorer, you can use a polyfill. Read the rest of this guide to learn how to roll your own, or you could skip it.
Creating Your Own Question Function for Parsing Strings
We should stick with the URL we used in the last section:
http://example.com/?product=shirt&color=blue&newuser&size=m
You can get all the URL arguments as a nice object with this function:
function getAllUrlParams(url) {
// get query string from url (optional) or window
var queryString = url ? url.split('?')[1] : window.location.search.slice(1);
// we'll store the parameters here
var obj = {};
// if query string exists
if (queryString) {
// stuff after # is not part of query string, so get rid of it
queryString = queryString.split('#')[0];
// split our query string into its component parts
var arr = queryString.split('&');
for (var i = 0; i < arr.length; i++) {
// separate the keys and the values
var a = arr[i].split('=');
// set parameter name and value (use 'true' if empty)
var paramName = a[0];
var paramValue = typeof (a[1]) === 'undefined' ? true : a[1];
// (optional) keep case consistent
paramName = paramName.toLowerCase();
if (typeof paramValue === 'string') paramValue = paramValue.toLowerCase();
// if the paramName ends with square brackets, e.g. colors[] or colors[2]
if (paramName.match(/\[(\d+)?\]$/)) {
// create key if it doesn't exist
var key = paramName.replace(/\[(\d+)?\]/, '');
if (!obj[key]) obj[key] = [];
// if it's an indexed array e.g. colors[2]
if (paramName.match(/\[\d+\]$/)) {
// get the index value and add the entry at the appropriate position
var index = /\[(\d+)\]/.exec(paramName)[1];
obj[key][index] = paramValue;
} else {
// otherwise add the value to the end of the array
obj[key].push(paramValue);
}
} else {
// we're dealing with a string
if (!obj[paramName]) {
// if it doesn't exist, create property
obj[paramName] = paramValue;
} else if (obj[paramName] && typeof obj[paramName] === 'string'){
// if property does exist and it's a string, convert it to an array
obj[paramName] = [obj[paramName]];
obj[paramName].push(paramValue);
} else {
// otherwise add the property
obj[paramName].push(paramValue);
}
}
}
}
return obj;
}
Soon, you’ll see how this works, but for now, here are some examples:
getAllUrlParams().product; // 'shirt'
getAllUrlParams().color; // 'blue'
getAllUrlParams().newuser; // true
getAllUrlParams().nonexistent; // undefined
getAllUrlParams('http://test.com/?a=abc').a; // 'abc'
You can try out this video as well.
Things You Should Know Before Using This Part
- As shown in the W3C specifications, our function thinks that the parameters are separated by the & symbol. But the style for URL parameters isn’t always clear, so you might see ; or & as separators sometimes.
- Some parameters may not have an equals sign or may have an equals sign but no value. Our code will still work.
- It stores the numbers of parameters that are used more than once in an array.
Now is the end of your search for a method that you could drop into your code. Read on to find out how the tool works.
You should already know about functions, objects, and arrays in JavaScript before reading this part. Check out the MDN JavaScript guide if you need to brush up on what you know.
How the Job Does It
It takes the URL’s query string (the part after the? and before the #) and turns it into a neat object with the data in it.
If we’ve given a URL, this line tells us to get everything after the question mark. If not, it tells us to just use the URL of the window:
var queryString = url ? url.split('?')[1] : window.location.search.slice(1);
After that, we’ll make an object to hold our parameters:
var obj = {};
We will begin to parse the question string if it exists. First, we need to get rid of the part that starts with #, since it’s not part of the question string:
queryString = queryString.split('#')[0];
We can now separate the question string into its parts:
var arr = queryString.split('&');
Next, we’ll go through this collection in a loop and separate each item into a key and a value. We’ll then add these to our object:
var a = arr[i].split('=');
First, let’s give each variable a key and a number. We’ll set it to true if there isn’t a parameter value to show that the parameter name exists. Yes, you can change this based on your needs:
var paramName = a[0];
var paramValue = typeof (a[1]) === 'undefined' ? true : a[1];
You can change all option names and values to lowercase if you want to. That way, someone won’t be able to send traffic to a URL with example=TRUE instead of example=true, which would cause your script to break. (This has happened to me.) You can leave this part out, though, if your query string needs to be case-sensitive:
paramName = paramName.toLowerCase();
if (typeof paramValue === 'string') paramValue = paramValue.toLowerCase();
The next thing we need to do is deal with the different kinds of information that can come in through paramName. It’s possible for this to be a regular string, an ordered array, or a non-indexed array.
If it’s a sorted array, we want the paramValue to also be an array with the value added at the right spot. If the array isn’t numbered, we want the value that goes with it to be an array with the element pushed on top of it. If the value is a string, we want to make a regular property on the object and assign the paramValue to it. If the property already exists, we want to turn the current paramValue into an array and add the new paramValue to it.
Just to show this, here is some example data and the result we would expect:
getAllUrlParams('http://example.com/?colors[0]=red&colors[2]=green&colors[6]=blue');
// { "colors": [ "red", null, "green", null, null, null, "blue" ] }
getAllUrlParams('http://example.com/?colors[]=red&colors[]=green&colors[]=blue');
// { "colors": [ "red", "green", "blue" ] }
getAllUrlParams('http://example.com/?colors=red&colors=green&colors=blue');
// { "colors": [ "red", "green", "blue" ] }
getAllUrlParams('http://example.com/?product=shirt&color=blue&newuser&size=m');
// { "product": "shirt", "color": "blue", "newuser": true, "size": "m" }
The last thing we do is return our object along with the numbers and parameters.
If your URL has any special letters encoded, like spaces (encoded as%20), you can also go this route to get back to the original value:
if (paramName.match(/\[(\d+)?\]$/)) {
var key = paramName.replace(/\[(\d+)?\]/, '');
if (!obj[key]) obj[key] = [];
if (paramName.match(/\[\d+\]$/)) {
var index = /\[(\d+)\]/.exec(paramName)[1];
obj[key][index] = paramValue;
} else {
obj[key].push(paramValue);
}
} else {
if (!obj[paramName]) {
obj[paramName] = paramValue;
} else if (obj[paramName] && typeof obj[paramName] === 'string'){
obj[paramName] = [obj[paramName]];
obj[paramName].push(paramValue);
} else {
obj[paramName].push(paramValue);
}
Just make sure you don’t decode something that has already been decoded, or your script will fail, especially if percents are there.
// assume a url parameter of test=a%20space
var original = getAllUrlParams().test; // 'a%20space'
var decoded = decodeURIComponent(original); // 'a space'
Anyway, good job! Thanks for reading this. You should now know how to get a URL field and have also learned some other useful skills.
In conclusion
Most of the time, the code in this piece will work when you get a URL query parameter. Always test and make changes based on what you find if you’re working with edge cases like rare separators or special formatting.
It’s possible to do more with URLs with the help of tools like query-string and Medialize URI.js. There are times when it makes more sense to use plain JavaScript, though, since it’s mostly just changing strings. Check everything to make sure it works for your needs, whether you write your own code or use a tool.
If you like playing around with strings, read our posts on how to split strings into substrings, change strings to numbers, and change numbers to strings and ordinals. Read our book JavaScript: Novice to Ninja, 2nd Edition to learn more about the language in depth.
How to Use JavaScript to Get URL Parameters
What do the terms in a web page mean?
Key-value pairs are added to the end of a URL. They are also called query parameters or query strings. There are “&” between them, and they’re usually used to send info from a web page to the server.
How can I use JavaScript to get to URL parameters?
To get to URL parameters, use the window.location.search property to get the whole query string. Then, use URLSearchParams or custom JavaScript functions to parse it and get to the parameters you want.
This is what window.location.search means in JavaScript.
The query string part of the URL can be found in the window.location.search object in the browser’s JavaScript environment. Everything after the “?” in the URL is part of it.
How do I use URLSearchParams to read URL parameters?
The URLSearchParams API gives you ways to get URL parameters and change them. With the query string, you can make a new instance of URLSearchParams and then use get() and other methods to get specific parameter values.
Is there a way to get URL values without using URLSearchParams?
Yes, you can use JavaScript methods like split() and forEach() to read the query string by hand. Using URLSearchParams, on the other hand, is easier and more consistent.